In this post, I’ll show you how to create a new user, modify an existing user and remove an old user in Windows Active Directory using PowerShell.
Create a Single User in Active Directory
The PowerShell New-ADUser CMDlet is used for creating a user in Active Directory.
PowerShell offers multiple ways to not only create a single user but to create Active Directory user objects in bulk. The CMDlet New-ADUser doesn’t have many mandatory parameters but you can use optional parameters while creating a new user.
- Using the OtherAttributes parameter, you can change property values that are not related to cmdlet parameters. The attribute name needs to be enclosed in single quotes when using this parameter.
- To create a user, you must give the SamAccountName parameter.
- The container or organizational unit (OU) for the new user is specified using the Path parameter. When the Path option is not used, the cmdlet creates a user object in the domain’s default user object container.
The following techniques describe various ways to build an object using this cmdlet:
- With the New-ADUser command, use the OtherAttributes parameter to specify the parameters and values and to set any additional values.
- A new user can be created from a template. Use the Instance parameter to create a new user or copy an existing one to the new object. The object used in the Instance parameter is used as a template.
- To create Active Directory user objects in bulk, combine the Import-Csv cmdlet with the New-ADUser cmdlet.
- Import a CSV file with a list of object properties to construct custom objects using the Import-Csv cmdlet.
- The New-ADUser cmdlet can then be used to construct user objects by passing these objects through its pipeline.
The following shows examples of the different parameters that can be used:
New-ADUser –SamAccountName “username” –DisplayName “username” –givenName “Username” –Surname “surname” –AccountPassword (ReadHost –AsSecureString “Message”) –Enabled $true –Path ‘CN=Users,DC=Doc,DC=Com’ –CannotChangePassword $false –ChangePasswordAtLogon $true –PasswordNeverExpires $false -EmailAddress “email” –EmployeeID “ID” –Department “string”
Below are the descriptions of parameters used in the above CMDlet:
After executing the command, PowerShell will ask for the password.
Enter the password and the user will be created.
Creating Bulk Users in Active Directory
Before creating the bulk users through PowerShell using the Import-CSV CMDlet, you will need to create a CSV file. The following is an example of the CSV file required:
Now, execute the following command to create bulk users in AD.
Import-CSV d:\Share\testing.csv | New-ADUser
The Import-CSV provides pipeline input to the New-ADUser CMDlet. It processes the values of the CSV file to create the new users. Executing this command will load the Active Directory module first.
After completing the action, you’ll return to the same prompt.
Check Active Directory for the newly created users.
Examples where Users would be Created with PowerShell
Here are some examples of where you may want to use PowerShell to add user accounts:
- Create a new user account.
- Create a user account in a particular OU.
- Create a user and set attributes not covered by the cmdlet’s parameters.
- Create an inetOrgPerson user.
- Create a new user based on an existing AD user.
- Create multiple user accounts using a CSV file.
- Create users in bulk using a PowerShell script.
- Create users in bulk by importing their attributes from a CSV file.
Create a New User Account
Specify only the account name.
The simplest example is creating a new user account by specifying only its name attribute:
New-ADUser M. Byrde
Running this will create the user but won’t show any output. To check whether the user was added successfully, we can list all Active Directory users using the following:
Get-ADUser -Filter * -Properties samAccountName | select samAccountName
However, the user we just created has more attributes than just a name; the following attributes are set by default:
- The account is created in the ‘Users’ container.
- The account is disabled.
- The account is a member of the Domain Users group.
- The user must reset the password at the first logon.
Note that many attributes are not populated including the password attribute meaning that no password is set
Specify Additional Attributes
To resolve this, we can a new account that is usable by specifying more attributes:
New-ADUser -Name "Ash Williams" -GivenName "Ash" -Surname "Williams" -SamAccountName "A.Williams" -UserPrincipalName "A.Williams@lpde4.local" -Path "OU=Managers,DC=lpde4,DC=com" -AccountPassword(Read-Host -AsSecureString "Input
The Read-Host parameter will ask you to input a new password. Note that the password should meet the length, complexity and history requirements of your domain security policy.
Let’s now see the results by running the following cmdlet:
Get-ADUser A.Williams -Properties CanonicalName, Enabled, GivenName, Surname, Name, UserPrincipalName, samAccountName, whenCreated, PasswordLastSet
To create a user account with even more attributes, use the following command:
New-ADUser -Name "Justin Hammer" -GivenName " Justin " -Surname " Hammer " -SamAccountName " Justin - Hammer " -AccountPassword (ConvertTo-SecureString -AsPlainText “webdir123R” -Force) -ChangePasswordAtLogon $True -Company "ABC Limited" -Title "CEO" -State "California" -City "San Francisco" -Description "Test Account Creation" -EmployeeNumber "45" -Department "Engineering" -DisplayName "Justin Hammer" -Country "US" -PostalCode "94001" -Enabled $True
To see a few of the new user’s attributes, use the following:
Get-ADUser -Identity Justin- Hammer -Properties * | select name,samaccountname,company,title,department,city,state,country,description,employeenumber,postalcode
Modify Users in Active Directory
Use the Set-ADUser CMDlet to modify the user in AD.
Set-ADUser –Identity “CN=TestUser7,CN=Users,DC=www,DC=DOC,DC=com” –SamAccountName “TestUser7” –LogonWorkStations “Test”
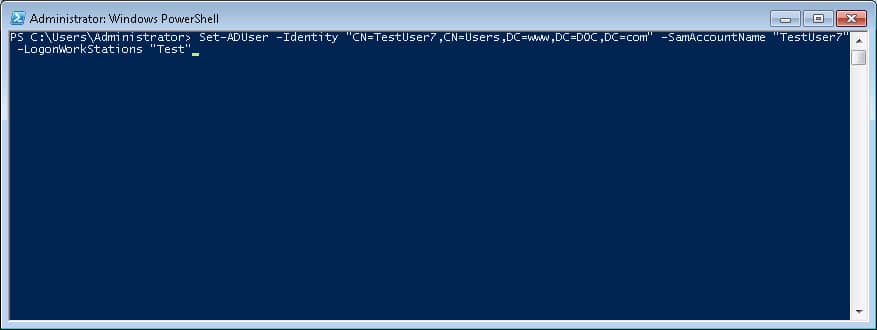
Some of the available parameters for this CMDlet are listed below.
Reset Password for AD Users
You can reset the password of a user with Set-ADAccountPassword CMDlet.
Set-ADAccountPassword –Identity “CN=TestUser7,CN=Users,DC=www,DC=DOC,DC=com” –SamAccountName “TestUser7” –LogonWorkStations “Test”
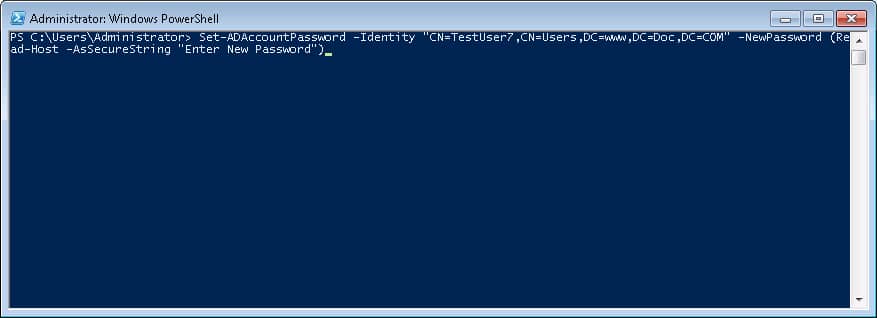
Some of the acceptable parameters for this CMDlet are listed below.
Removing a Active Directory User Account
You can remove a user account using the Remove-ADUser CMDlet.
Remove-ADUser –Identity “CN=Username,CN=Users,DC=doc,DC=com”
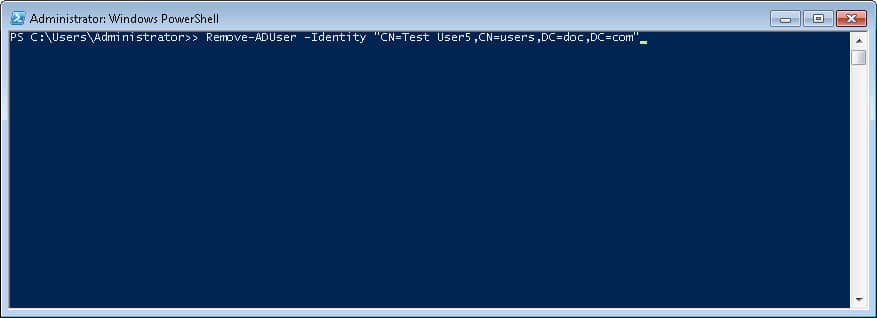
Pressing the Enter key will ask for confirmation to delete the user.
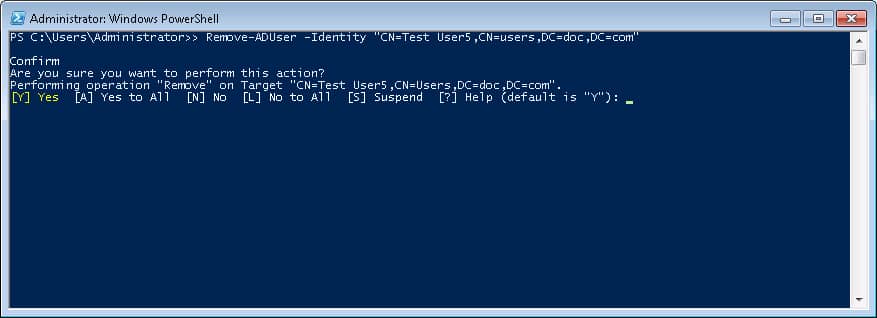
Press Y to confirm the action.
Create an inetOrgPerson User
Most user objects in Active Directory have the class of user. But you can also create user objects with the class inetOrgPerson, which has user as a parent class. The inetOrgPerson class facilitates integration with certain applications and simplifies the migration of certain user objects into Active Directory.
To create an inetOrgPerson user account, simply include the -Type parameter and specify inetOrgPerson as its value:
New-ADUser -Name "Neal Gamby" -Path "OU=NBC,DC=LPDE4,DC=local" -GivenName "nEAL" -Surname " Gamby " -SamAccountName "Neal.Gamby " -AccountPassword (ConvertTo-SecureString -AsPlainText “webdir123R” -Force ) -ChangePasswordAtLogon $True -DisplayName "Neal Gamby" -Enabled $True -Type iNetOrgPerson
Conclusion
To ensure security of your Active Directory it is important to keep track of user creation, modification and deletion activities. With native methods, it is very difficult to monitor these activities. Lepide Active Directory Auditor can help you to audit user creation, modification and deletion in real time with other important AD changes.